Introduction
Python is one of the most popular programming languages in the world, renowned for its simplicity and versatility. If you’re preparing for a Python-related job interview, it’s crucial to familiarize yourself with common coding questions and concepts. This comprehensive guide will help you master the essential Python interview coding questions, boosting your confidence and chances of success.
Table of Contents
- Introduction
- Common Python Interview Coding Questions
- Advanced Python Coding Questions
- Coding Exercises and Solutions
- Conclusion
- Key Takeaways
- FAQs
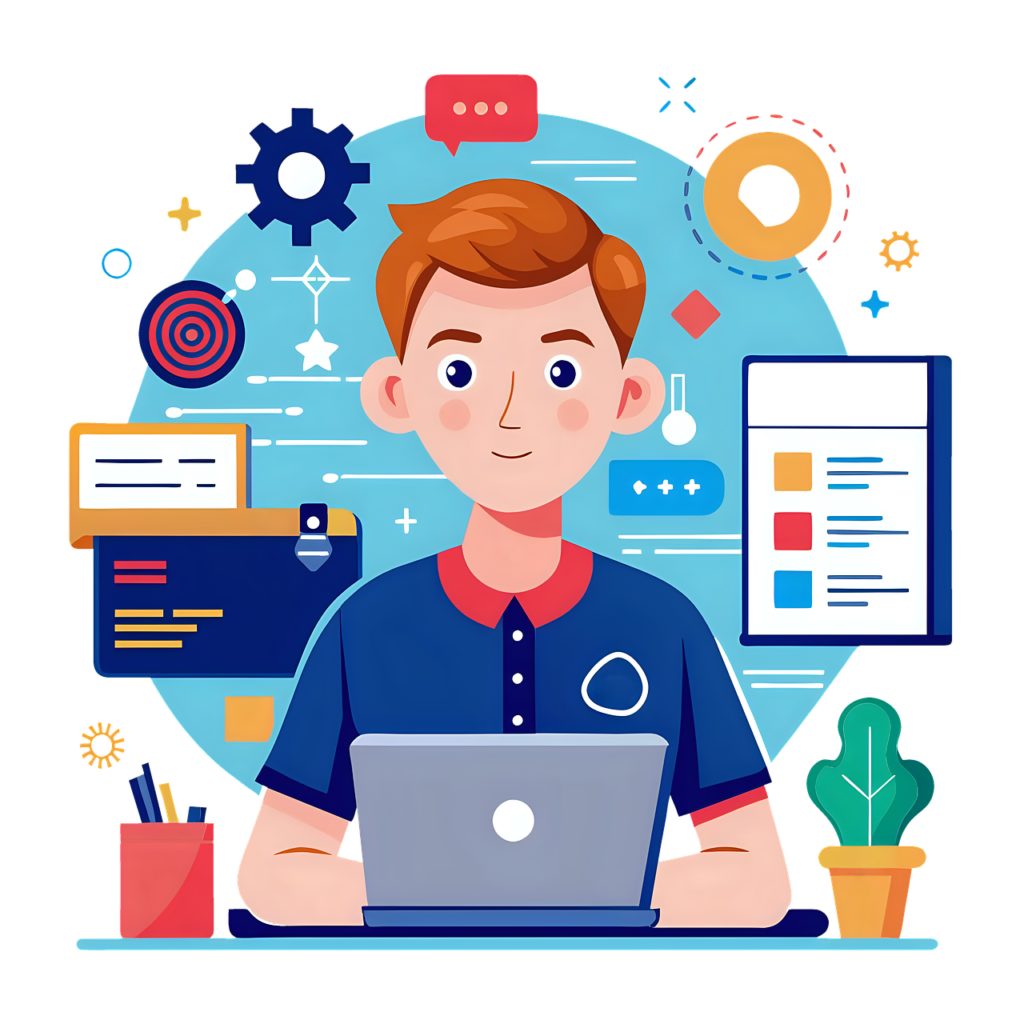
Common Python Interview Coding Questions
1. What is Python?
Python is a high-level, interpreted programming language known for its readability and simplicity. It supports multiple programming paradigms, including procedural, object-oriented, and functional programming. Python is widely used in web development, data analysis, artificial intelligence, scientific computing, and more.
2. Explain the difference between Python 2 and Python 3.
Python 2 and Python 3 are two major versions of Python. Python 3 introduced several improvements and changes over Python 2, such as:
- Print Function: In Python 3,
print
is a function, whereas in Python 2, it’s a statement. - Integer Division: In Python 3, division of integers using
/
results in a float, whereas in Python 2, it results in an integer. - Unicode: Python 3 uses Unicode by default for string representation, enhancing support for internationalization.
3. How do you manage memory in Python?
Python manages memory using a built-in garbage collector that automatically deallocates memory when objects are no longer in use. The garbage collector uses reference counting and cyclic garbage collection to identify and collect unused objects.
4. What are Python decorators?
Decorators in Python are functions that modify the behavior of other functions or methods. They are used to add functionality to existing code in a concise and readable manner. Decorators are applied using the @decorator_name
syntax above the function definition.
5. What is the purpose of the self
keyword in Python classes?
The self
keyword in Python is used to refer to the instance of the class within its methods. It allows access to the attributes and methods of the class. Using self
, you can define instance variables and methods that operate on the specific instance of the class.
Advanced Python interview Coding Questions
1. Explain the concept of list comprehensions with an example.
List comprehensions provide a concise way to create lists in Python. They consist of an expression followed by a for
clause, and can also include optional if
clauses. For example:
pythonCopy code# Create a list of squares of even numbers from 0 to 9
squares = [x**2 for x in range(10) if x % 2 == 0]
print(squares) # Output: [0, 4, 16, 36, 64]
2. What are Python generators and how do they differ from iterators?
Generators are a special type of iterator that allow you to iterate over a sequence of values lazily, meaning they generate values on the fly and don’t store them in memory. They are defined using the yield
keyword. Unlike regular functions, generators return an iterator object.
pythonCopy codedef fibonacci(n):
a, b = 0, 1
while a < n:
yield a
a, b = b, a + b
# Create a generator for Fibonacci numbers up to 100
fib_gen = fibonacci(100)
for num in fib_gen:
print(num)
3. How do you handle exceptions in Python?
Exceptions in Python are handled using try
, except
, else
, and finally
blocks. The try
block contains code that might raise an exception, and the except
block contains code to handle the exception. The else
block contains code that runs if no exceptions are raised, and the finally
block contains code that always runs, regardless of whether an exception occurred.
pythonCopy codetry:
result = 10 / 0
except ZeroDivisionError:
print("Cannot divide by zero")
else:
print("Division successful")
finally:
print("Execution complete")
4. What are Python’s built-in data structures?
Python has several built-in data structures, including:
- Lists: Ordered, mutable collections of items.
- Tuples: Ordered, immutable collections of items.
- Sets: Unordered collections of unique items.
- Dictionaries: Unordered collections of key-value pairs.
5. Explain the Global Interpreter Lock (GIL) in Python.
The Global Interpreter Lock (GIL) is a mutex that protects access to Python objects, preventing multiple threads from executing Python bytecode simultaneously. This ensures thread safety but can be a limitation in CPU-bound multi-threaded programs. To overcome this, Python provides alternatives like multiprocessing.
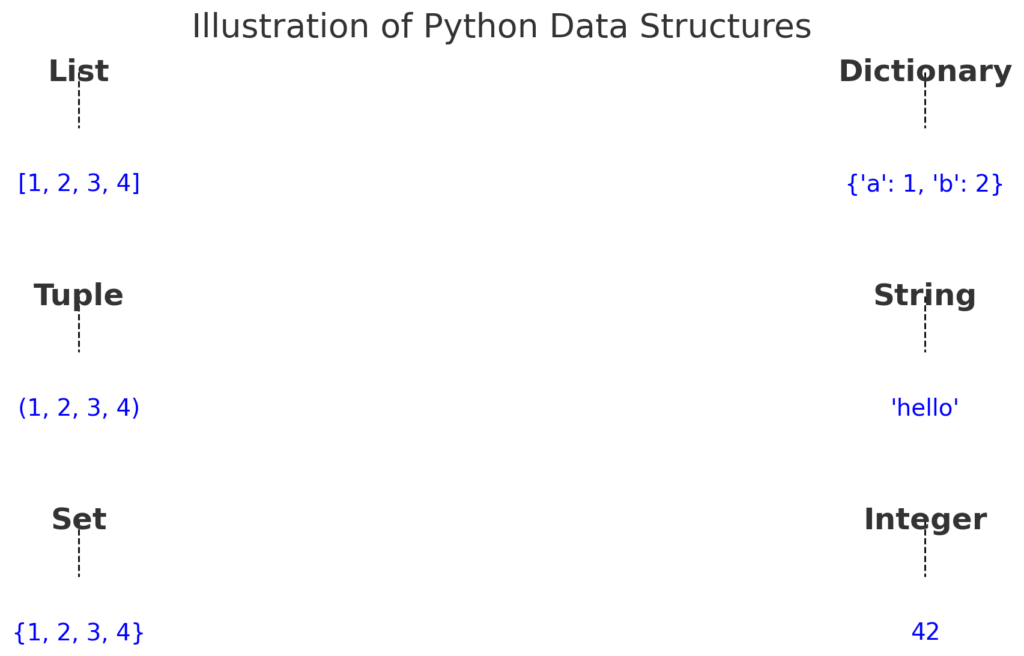
Coding Exercises and Solutions
Exercise 1: Reverse a String
Write a function to reverse a given string.
pythonCopy codedef reverse_string(s):
return s[::-1]
# Test the function
print(reverse_string("hello")) # Output: "olleh"
Exercise 2: Check for Anagrams
Write a function to check if two strings are anagrams of each other.
pythonCopy codedef are_anagrams(str1, str2):
return sorted(str1) == sorted(str2)
# Test the function
print(are_anagrams("listen", "silent")) # Output: True
print(are_anagrams("hello", "world")) # Output: False
Exercise 3: Find the Largest Element in a List
Write a function to find the largest element in a list.
pythonCopy codedef find_largest(lst):
return max(lst)
# Test the function
print(find_largest([1, 2, 3, 4, 5])) # Output: 5
Exercise 4: Remove Duplicates from a List
Write a function to remove duplicates from a list while preserving the order.
pythonCopy codedef remove_duplicates(lst):
seen = set()
result = []
for item in lst:
if item not in seen:
seen.add(item)
result.append(item)
return result
# Test the function
print(remove_duplicates([1, 2, 2, 3, 4, 4, 5])) # Output: [1, 2, 3, 4, 5]
Exercise 5: Fibonacci Sequence
Write a function to generate the Fibonacci sequence up to n
terms.
pythonCopy codedef fibonacci_sequence(n):
fib = [0, 1]
while len(fib) < n:
fib.append(fib[-1] + fib[-2])
return fib[:n]
# Test the function
print(fibonacci_sequence(10)) # Output: [0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
Conclusion
Preparing for a Python interview coding questions requires a solid understanding of both basic and advanced concepts, as well as the ability to solve coding challenges efficiently. By mastering these common and advanced Python interview coding questions, you’ll be well-equipped to tackle your next interview with confidence.
Key Takeaways
- Python is a versatile language used in various fields such as web development, data analysis, and scientific computing.
- Understanding the differences between Python 2 and Python 3 is crucial.
- Memory management in Python is handled by a built-in garbage collector.
- Decorators and generators are powerful features that enhance Python’s functionality.
- Exception handling is essential for writing robust code.
- Familiarize yourself with Python’s built-in data structures.
- Practice coding exercises to reinforce your knowledge and improve your problem-solving skills.
FAQs
Q1: What are some common topics covered in Python interview coding questions?
Common topics include data structures (lists, tuples, dictionaries, sets), algorithms (sorting, searching), object-oriented programming, exception handling, and Python-specific features like decorators and generators.
Q2: How can I improve my Python coding skills?
Practice regularly by solving coding challenges on platforms like LeetCode, HackerRank, and CodeSignal. Additionally, contribute to open-source projects and review other people’s code to learn new techniques.
Q3: What is the best way to prepare for a Python interview coding questions?
Start by reviewing the basics of Python and then move on to more advanced topics. Practice coding problems, understand common interview questions, and work on projects to build a strong portfolio.
Q4: Are there any specific Python libraries I should know for python interview coding questions?
Familiarity with libraries like NumPy, Pandas, and Matplotlib can be beneficial, especially for data analysis and visualization roles. For web development roles, knowledge of Django or Flask is useful.
Q5: How important is it to know the differences between Python 2 and Python 3?
While most modern applications use Python 3, some legacy systems may still use Python 2. Understanding the differences can help you adapt to various environments and codebases.
More about python interview coding questions: https://www.analyticsvidhya.com/blog/2022/07/python-coding-interview-questions-for-freshers/
More Blogs: https://scriptedkiddies.com